The apply
, lapply
, sapply
, mapply
, and tapply
functions in R are part of the apply family, which provides a concise way to perform operations on data structures without the need for explicit loops. These functions are great for repetitive tasks, enhancing readability, and reducing code length.
1. apply()
Applies a function to rows or columns of a matrix or data frame. The syntax is: apply(X, MARGIN, FUN, …) where X
: The input matrix or data frame; MARGIN
: 1 for rows, 2 for columns; FUN
: The function to apply.
apply(mtcars, 2, mean)
# Calculate the mean of each column.
2. lapply()
Applies a function to each element of a list or vector and returns the result as a list. The syntax is: lapply(X, FUN, …) where X
: The input list or vector; FUN
: The function to apply.
lapply(mtcars, mean)
# Compute the mean of each column and return a list.
3. sapply()
A simplified version of lapply()
. It applies a function to each element of a list or vector but tries to simplify the output (e.g., a vector or matrix instead of a list). The syntax is: sapply(X, FUN, …).
sapply(mtcars, mean)
# Compute the mean of each column and return a vector.
4. mapply()
A multivariate version of sapply()
. It applies a function to multiple arguments simultaneously. The syntax is: mapply(FUN, …, MoreArgs = NULL).
prod_mpg_hp <- mapply(*, mtcars$mpg, mtcars$hp)
# Multiply mpg and hp for each row.
print(prod_mpg_hp)
5. tapply()
Applies a function to subsets of a vector defined by a grouping factor or factors. The syntax is: tapply(X, INDEX, FUN, …) where X
: The input vector; INDEX
: The grouping factor(s); FUN
: The function to apply.
tapply(mtcars$mpg, mtcars$cyl, mean)
# Calculate the mean mpg by the number of cylinders (cyl).
Comparison of Functions
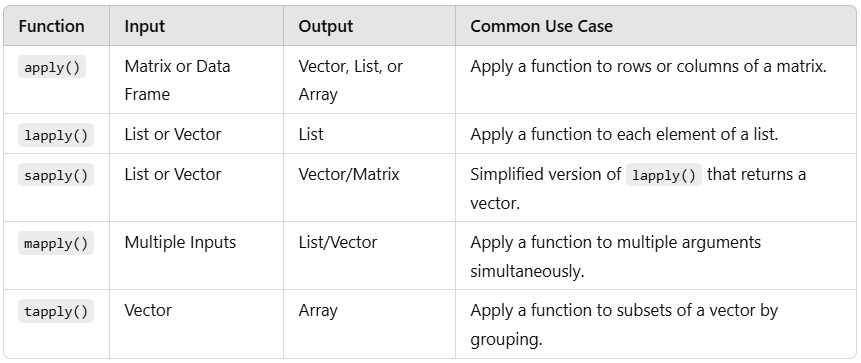